Combobox
A powerful dropdown for providing suggestions, filtering out large datasets, and creating new values quickly and efficiently with no overhead.
Overview
Loading...
Loading...
Resources
Install
yarn add @activecampaign/camp-components-combobox
Variations
A combobox is a combination of input and dropdown components.
The dropdown enables a user to select an option from the dataset presented inside a popup and may contain a listbox, a grid (such as a calendar), or a custom representation of the dataset. The input is used for entering a value, which can either filter out options inside a limited dataset or create a new entry in the dataset.
Single select combobox
The Single Select combobox can have a controlled
or uncontrolled
state. This is true for both variants (editable
and filterable
).
Uncontrolled state
Example implementation of the editable variant with uncontrolled state:
// this is the type of the option data that we are using in this example
export interface ICharacter {
name: string;
franchise: string;
}
/**
* function for filtering the 'options' data
* (taken from downshifts documentation, example of doing a client side search)
* @param inputValue
*/
function filterOptions(inputValue?: string) {
const lowerCasedInputValue = inputValue?.toLowerCase();
return function booksFilter(option: ICharacter) {
return (
!inputValue ||
(lowerCasedInputValue && option?.name?.toLowerCase().includes(lowerCasedInputValue)) ||
(lowerCasedInputValue && option?.franchise?.toLowerCase().includes(lowerCasedInputValue))
);
};
}
export const EditableUncontrolled = () => {
const [options, setOptions] = React.useState(smash_characters);
const strings = options.map(({ name }) => name);
return (
<Combobox
inputAriaLabel="Choose your character"
options={strings}
variant="editable"
optionToString={(option) => option?.value as string}
onCreate={(value) => console.log('onCreate fired:', value)}
onInputValueChange={({ inputValue }) =>
setOptions(smash_characters.filter(filterOptions(inputValue)))
}
/>
);
};
Controlled state
To control the input value, use the inputValue
prop. Then, set the change handler prop onInputValueChange
to the function you would like to use when the input value changes.
Example implementation of the filterable variant with controlled state:
// this is the type of the option data that we are using in this example
export interface ICharacter {
name: string;
franchise: string;
}
/**
* function for filtering the 'options' data
* (taken from downshifts documentation, example of doing a client side search)
* @param inputValue
*/
function filterOptions(inputValue?: string) {
const lowerCasedInputValue = inputValue?.toLowerCase();
return function booksFilter(option: ICharacter) {
return (
!inputValue ||
(lowerCasedInputValue && option?.name?.toLowerCase().includes(lowerCasedInputValue)) ||
(lowerCasedInputValue && option?.franchise?.toLowerCase().includes(lowerCasedInputValue))
);
};
}
export const FilterableControlled = () => {
const [options, setOptions] = React.useState(smash_characters); // options array
const strings = options.map(({ name }) => name); // convert 'options' to strings array for this example
const [value, setValue] = React.useState('');
return (
<>
<Text.Heading>controlled state: {value}</Text.Heading>
<Combobox
inputAriaLabel="Choose your character"
options={strings}
inputValue={value}
variant="filterable"
optionToString={({ value }) => value as string}
onNoResults={() => setValue('')}
onSelect={(option) => option && setValue(option)}
onInputValueChange={({ inputValue }) => {
setValue(inputValue as string);
setOptions(smash_characters.filter(filterOptions(inputValue)));
}}
/>
</>
);
};
Multiselect combobox
Unlike the Single Select combobox, the Multiselect combobox must have a controlled state in order to track the options the user has selected.
Required props for multiselect
deselectOption
Callback function to remove a selected option from multiselect comboboxmultiselect
Set to true for multiselect comboboxselectedOptions
The current array of selected options as strings
// this is the type of the option data that we are using in this example
export interface ICharacter {
name: string;
franchise: string;
}
/**
* function for filtering the 'options' data
* (taken from downshifts documentation, example of doing a client side search)
* @param inputValue
*/
function filterOptions(inputValue?: string) {
const lowerCasedInputValue = inputValue?.toLowerCase();
return function booksFilter(option: ICharacter) {
return (
!inputValue ||
(lowerCasedInputValue && option?.name?.toLowerCase().includes(lowerCasedInputValue)) ||
(lowerCasedInputValue && option?.franchise?.toLowerCase().includes(lowerCasedInputValue))
);
};
}
export const MultiselectCombobox = () => {
// initialize state with an array of data representing the options in your combobox
const [currentOptions, setCurrentOptions] = React.useState(options_array);
// we are converting the options to an array of strings in this example
const strings = currentOptions.map(({ name }) => name);
// initialize input value state
const [value, setValue] = React.useState('');
// initialize selected value state
const [selected, setSelected] = React.useState<string[]>([]);
// this is the function called when a chip's dismiss button is clicked
// removes the option from the selected state
const handleDeselectOption = (optionToRemove) => {
const newSelected = selected.filter((option) => option !== optionToRemove);
setSelected(newSelected);
};
return (
<Combobox
// multiselect must have a controlled input
inputValue={value}
options={strings}
// you may also use filterable variant for multiselect
variant="editable"
optionToString={(option) => option?.value as string}
// onCreate is fired when the user clicks to add a new value that doesn't
// already exist in the options provided
onCreate={(value) => console.log('onCreate fired:', value)}
// this function currently allows you to select duplicates but you can add validation
// here to avoid that
onSelect={(item) => {
if (item) {
// reset input to empty
setValue('');
// reset items in menu to all items
setCurrentOptions(options_array);
// check if item is already selected
const isAlreadySelected = selected.filter(option => option === item)
// add selected item to array
const newSelected = isAlreadySelected.length > 0 ? [...selected] : [...selected, item];
setSelected(newSelected);
}
}}
onInputValueChange={({ inputValue }) => {
setValue(inputValue as string);
// see filterOptions function defined above
setCurrentOptions(options_array.filter(filterOptions(inputValue)));
}}
multiselect
// selectedOptions should be an array of strings
// strings should match the results from optionToString function
selectedOptions={selected}
deselectOption={handleDeselectOption}
label="Tag to be added"
placeholder="Enter tag"
/>
);
};
Usage
Props
variant
Use the editable
variant to allow the user to create new items based on their input into the combobox. For example, if they type a tag value that doesn’t already exist in their list of tags, you may give them the option to create their value as a new tag. Use this in combination with the onCreate
prop to specify the callback function which creates the new item.
Use the filterable
variant when the user is only allowed to search among preexisting options. If no option exists that matches their input value, the user will see a “No results” message - for example, when a user is searching for a contact name and the contact isn’t in their list. If the user clicks on the “No results” message in the dropdown, the input value is cleared and the onNoResults
callback, if provided via props, is called.
optionToString
The optionToString
prop determines what text is rendered within the list options in the menu. If your combobox accepts a list of strings, you will simply need to return the callback argument like so:
optionToString={(option: string) => option}
If your combobox accepts a list of objects, you will need to specify which value to render within the list option. You may need to do something like the below:
export const Objects: StoryFn<ComboboxProps<ICharacter>> = () => {
const [options, setOptions] = React.useState(smash_characters);
return (
<Combobox<ICharacter>
inputAriaLabel="Choose your character"
options={options}
variant="filterable"
renderOption={({ value }) => value?.name}
optionToString={(option: { value: { name: string } }) => option?.value?.name}
onInputValueChange={({ inputValue }) =>
setOptions(smash_characters.filter(filterOptions(inputValue)))
}
/>
);
};
renderOption
Render callback for rendering content of option in list; not needed if you’re rendering a list of strings. The combobox supports the ability to render additional JSX within the list options via a renderOption
callback. This is particularly useful when working with objects, as there may be additional fields that are part of the object you want to display within your option.
export const CustomRenderOptions: StoryFn<ComboboxProps<ICharacter>> = () => {
const [options, setOptions] = React.useState(smash_characters);
return (
<Combobox<ICharacter>
inputAriaLabel="Choose your character"
options={options}
variant="filterable"
optionToString={(option) => option?.value?.name as string}
renderOption={({ value }) => {
return (
<div>
<div style={{ fontSize: '0.75rem' }}>
<span
style={{
borderBottom: '2px solid darkgray',
}}
>
{value?.name}
</span>
</div>
<small style={{ fontSize: '0.7rem', color: 'gray' }}>{value?.franchise}</small>
</div>
);
}}
onInputValueChange={({ inputValue }) =>
setOptions(smash_characters.filter(filterOptions(inputValue)))
}
/>
);
};
Best practices
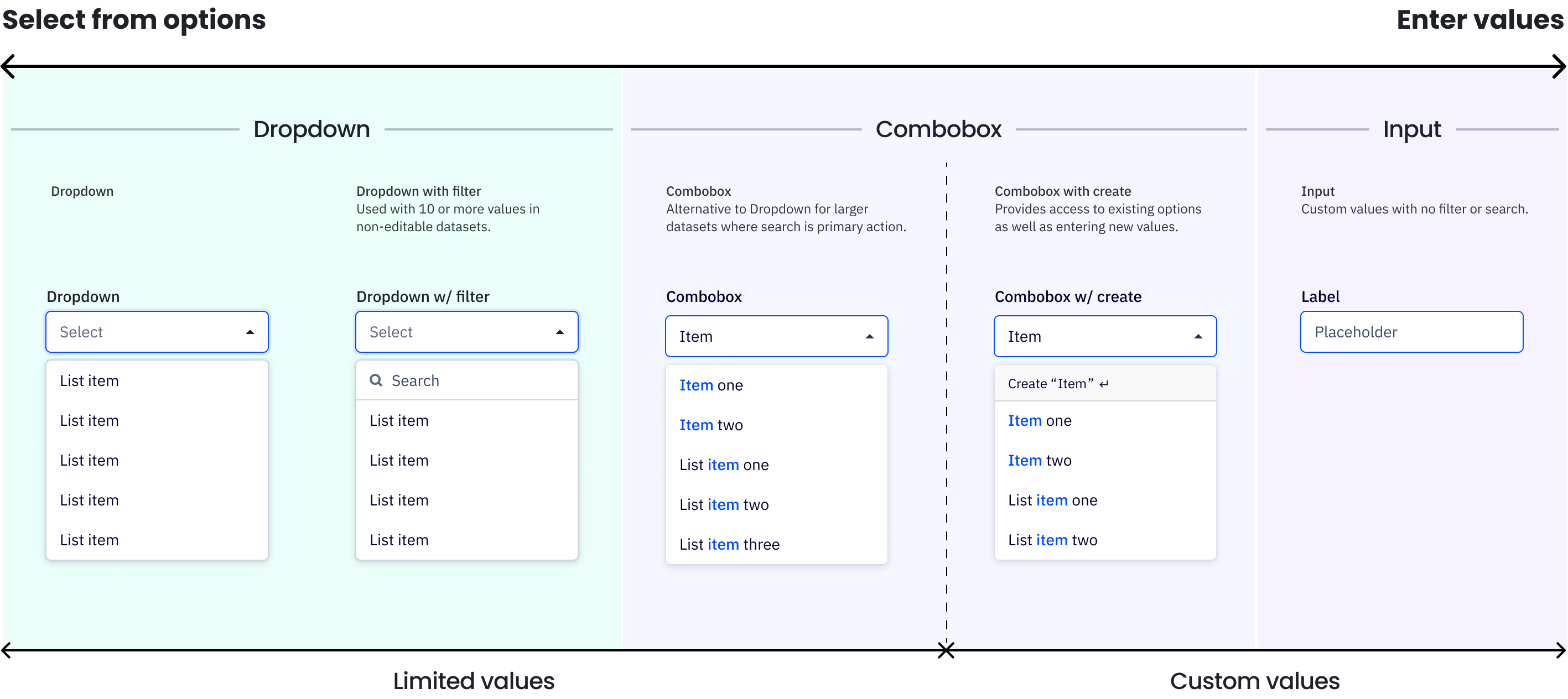
The primary action when using a dropdown is selecting an option from the list, while for the combobox it’s searching for the right option. A small dataset should use a dropdown without a filter, a medium dataset can add a search field to the dropdown to filter results (i.e. a list of campaigns), and large to infinite datasets (i.e. mail addresses) are a better fit for the comboboxes.
✅ DO
- Do use in large data sets where a user is more likely to search for their option by typing than scrolling.
🚫 DON’T
- Don’t use for small datasets where filtering is not needed, such as 15 items or less.
Accessibility
Keyboard support
- To open the combobox on focus, press
space
to open the menu or start typing to search the menu - When the dropdown menu is open, use
↑
or↓
to navigate andenter
to select an item within the list - In an editable combobox, if a user is inputting a custom input, pressing
enter
will save that input and create it as a new item Tab
or (shift
+tab
to move backward) closes the combobox and moves focus